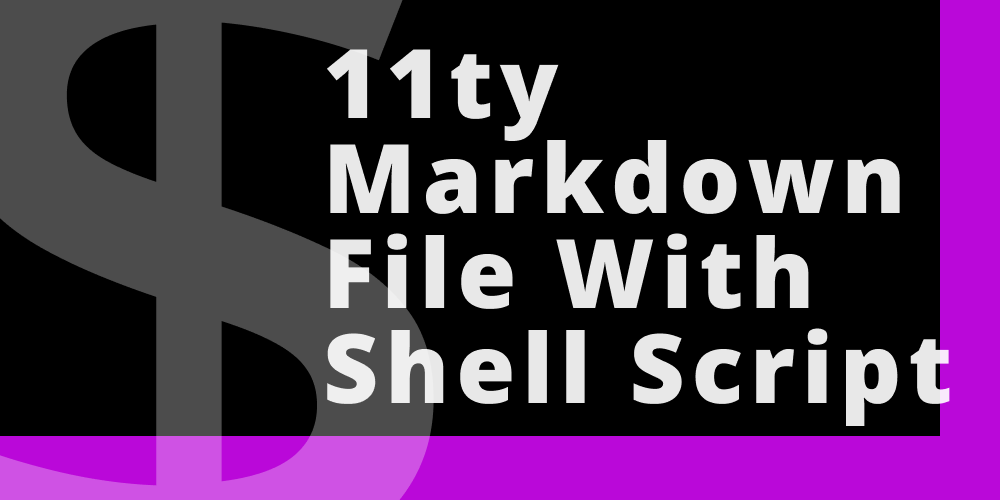
I have been using Save As to create new web pages from old ones for my blog for some time now. This works okay, but I have to rename the file, delete out the old content, then leave some of the reusable HTML snippets like images, links and resources, which can be a bit cumbersom. So to automate this process, I created a shell script that creates a markdown file and uses the title name as the file name and adds all my reusable snippets.
To do this, create a shell script file and keep it somewhere convenient to output a ready to go 11ty markdown file. This spawns a new file using the command line tool and adds the yaml front matter as well as anything else that gets use on a regular basis. I added HTML snippets for images, external links and the bottom of page references, that I usually use in every post.
Here's what the template file look's like, that the script file creates.
---
title: Template for Creating Post
date: 2023-07-31
description: Post description here.
---
<a href="#" target="_blank" rel="noopener">This is an external link</a>
<img src="/assets/blog/category/name-of-image.jpg" class="center shadow" alt="" width="418" height="auto">
<h2>Resources</h2>
<ul>
<li><a href="#" target="_blank" rel="noopener">Resource</a></li>
<li><a href="#" target="_blank" rel="noopener">Resource</a></li>
</ul>
This is a simplified version of what I use, as I have a bunch more custom front matter in each post, like post image, image alt, highlighting script ext...
It makes creating new formatted files more consistant and easier to manage as the content grows and changes.
Bash Shell
You will need Bash installed on your machine. If you have a Windows PC you can use Git Bash and be good to go. If you have a Mac, Bash is already included in all versions as it is a linux based software.
How to create a Shell Script
First create a file with the .sh
extension like create-markdown.sh
. Then add #! /bin/bash
that is known as shebang which is a combination of bash #
and bang !
followed by the bash shell path. It is always the first line of the script. The Shebang tells the shell to execute via the bash shell. This is the absolute path to the bash interpreter.
Put all the front matter and reusable HTML snippets in a generate_output
function. Use $1
varible to store the title. We'll add the title when running the file, then use the title to create the file name.
generate_output() {
echo "title: $1" # Pass the title as the first argument to the script
}
Then use an if statement to check that the title was added when running the file. This will serve as a remider how to run the script if it is ran wrong. The \
back slashes are used to excape the quotes.
# Check if an argument (title) is provided
if [ -z "$1" ]; then
echo "Usage: sh create_markdown.sh \"title\""
exit 1
fi
Get the title from the first argument title="$1"
. Then lowercase and replace the title spaces with dashes to create the filename.
filename=$(echo "$title" | tr '[:upper:]' '[:lower:]' | tr ' ' '-')
Then redirect the generate_output
function content to the $output_file
that equals the filename variable, and was created from the title name. Finally echo out a prompt to say that the file and snippets have been created!
Final code.
#!/bin/bash
# Function to create YAML front matter and reusable HTML
generate_output() {
echo "---"
echo "title: $1" # Pass the title as the first argument to the script
echo "date: $(date +"%Y-%m-%d")" # Use the current date as the "date" field in the front matter
echo "description: Post description here."
echo "---"
echo
echo
echo
echo
echo "<a href=\"#\" target=\"_blank\" rel=\"noopener\">This is an external link</a>"
echo
echo "<img src=\"/assets/blog/category/name-of-image.jpg\" class=\"center shadow\" alt=\"\" width=\"418\" height=\"auto\">"
echo
echo "<h2>Resources</h2>
<ul>
<li><a href=\"#\" target=\"_blank\" rel=\"noopener\">Resource</a></li>
<li><a href=\"#\" target=\"_blank\" rel=\"noopener\">Resource</a></li>
</ul>"
}
# Check if an argument (title) is provided
if [ -z "$1" ]; then
echo "Usage: ./create_markdown.sh [title]"
exit 1
fi
# Get the title from the first argument
title="$1"
# Lowercase and replace spaces in the title with dashes to create the filename
filename=$(echo "$title" | tr '[:upper:]' '[:lower:]' | tr ' ' '-')
# Create the Markdown file
output_file="$filename.md"
generate_output "$title" > "$output_file"
echo "Markdown file '$output_file' with YAML front matter and HTM snippets created successfully!"
To run the script open the command line for Mac or Git Bash for Windows in the folder that you saved the file in. Then run sh create-markdown.sh "The Title of the Post"
. This will output your new file in this folder.
Final Thoughts
One of the things that I really like about 11ty and the jamstack is that it is a break from what can be UI madness when working with WordPress. It allows you to work directly in the files on your machine. Instead of clicking through a slow loading interface that can easily get bogged down with UI options that your not using.
The drawback to this is that you now are responsible for keeping everthing organized in a way that will allow you to scale. That is where using automated scripts and also taking advantage of GitHub will help for processes like post creation and organization. Expecially if you are in a team environment.