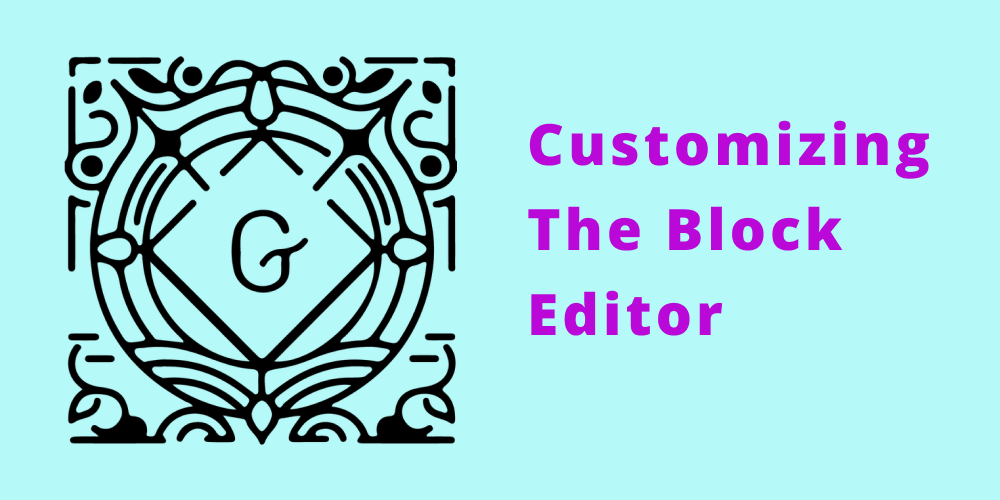
Out of the box Gutenberg comes with many different types of blocks, which is nice. Although if you are wanting a more curated editor UI, than we need to limit what is getting loaded here.
Gutenberg Block Manager
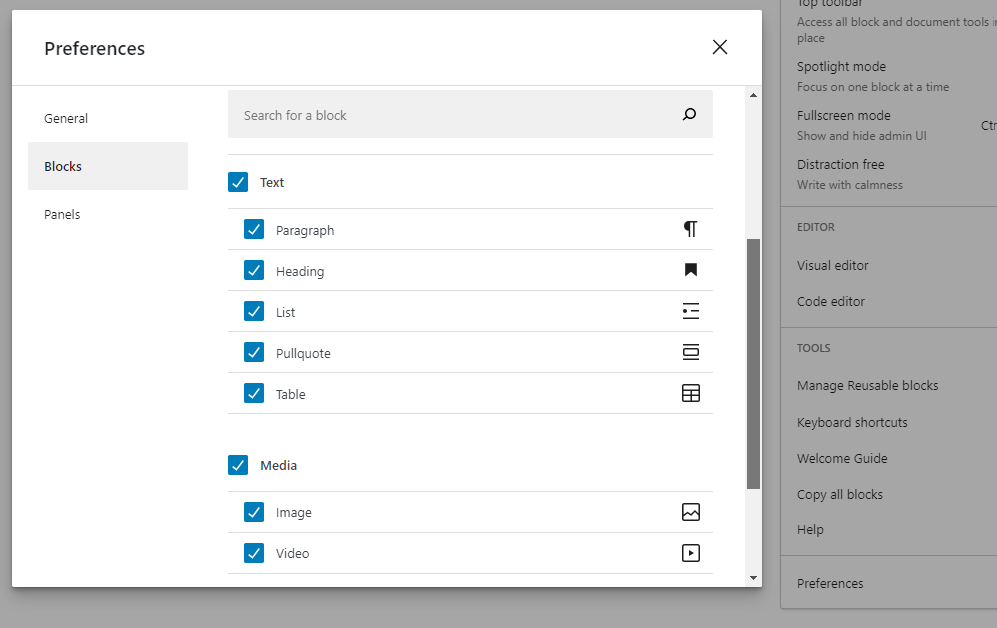
The first option is to use the UI and go to the View settings located in the top right three dots of the editor post header and then to Preferences. From there in the Blocks tab you can choose which block you want to be visible.
Manage via custom plugin
If you need more control over the Gutenberg UI, I recommend creating a WordPress plugin to house your Gutenberg customizations. So first we will start with creating a standard WordPress plugin.
Create a folder in the wp-content/plugin
folder with the name of your plugin like my-gutenberg
. Then create a php
file with the name of my-gutenberg.php
.
<?php
/**
* Plugin Name: My Gutenberg
* Description: Gutenberg customizations
* Version: 0.1.0
* Author: Jeremy Faucher
* Text Domain: my-gutenberg
*/
Only the plugin’s name is required, but if you intend to distribute your plugin, you should add as much data as possible.
Next we can go to the admin > Plugins and > Activate our new plugin. The plugin doesn’t do anything yet, but it is now an active and a functioning plugin.
allowed_block_types_all
Now we can add the WordPress allowed_block_types_all
filter to the plugin. By default this filter will white list all the blocks and return a emty array. So we need to add the WordPress core slugs of the blocks we want to show.
/**
* Only allow specific Gutenberg core blocks
*/
function allowed_block_types() {
return array(
'core/paragraph',
'core/heading',
'core/list',
'core/list-item',
'core/table',
'core/pullquote',
'core/image',
'core/embed',
'core/video',
'core/block'
);
}
add_filter( 'allowed_block_types_all', 'allowed_block_types', 25, 2 );
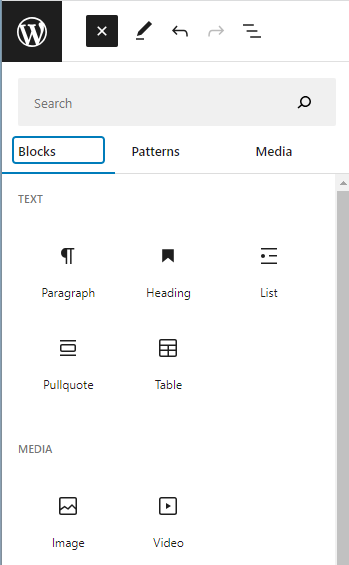
This array has the more standard blocks that we typically use and you can see a complete list of core blocks in the WordPress reference guide. The core/block
gives you a reusable block feature that allows you to create custom reusable components.
Embed Blocks
To limit the embed blocks, first we need to allow all of them to show by adding the above core/embed
slug to the allowed_block_types_all
filter. Then via JavaScript file limit which of the embed blocks to show.
First create a JavaScript file called my-block-filter.js
in your plugin folder. In your plugin my-gutenberg.php
file enqueue the file using the enqueue_block_editor_assets
action hook. Then give it the parameters of wp-blocks
, wp-dom-ready
and wp-edit-post
.
/**
* Enqueue scripts and styles
*/
function my_gutenberg_scripts() {
wp_enqueue_script(
'my-block-filters',
plugin_dir_url( __FILE__ ) . 'my-block-filters.js',
array( 'wp-blocks', 'wp-dom-ready', 'wp-edit-post' )
);
}
add_action( 'enqueue_block_editor_assets', 'my_gutenberg_scripts' );
Now create a my-block-filter.js
file in the plugin folder.
Using the Block Variations API we can use the unregisterBlockVariation()
function to only show the ones we want. Here create an array of embed blocks you want to show, then use the indexOf
method in a forEach
loop to see if the other blocks match the array we created. Now we can unregister the other blocks that are not in the array from the core/embed
slug.
wp.domReady( () => {
/**
* Only allow the following embeds
*
*/
const allowedEmbedBlocks = [
'youtube',
'vimeo',
];
wp.blocks.getBlockVariations('core/embed').forEach(function (blockVariation) {
if (-1 === allowedEmbedBlocks.indexOf(blockVariation.name)) {
wp.blocks.unregisterBlockVariation('core/embed', blockVariation.name);
}
});
} );
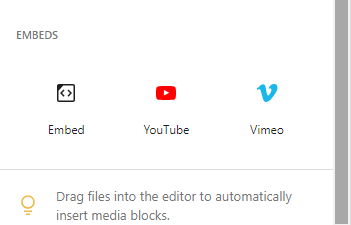
The block editor’s data
To trouble shoot issues you can use the wp.data.select
for retriving data from the application state. To get a list a all the allowed block types we can check the data stored in the core/block-editor
slug.
wp.data.select('core/block-editor').getSettings().allowedBlockTypes;
Run this is the console of the editor page to see a list of the allowed block types. Go to the block editor’s data to see a list of all the selector options.
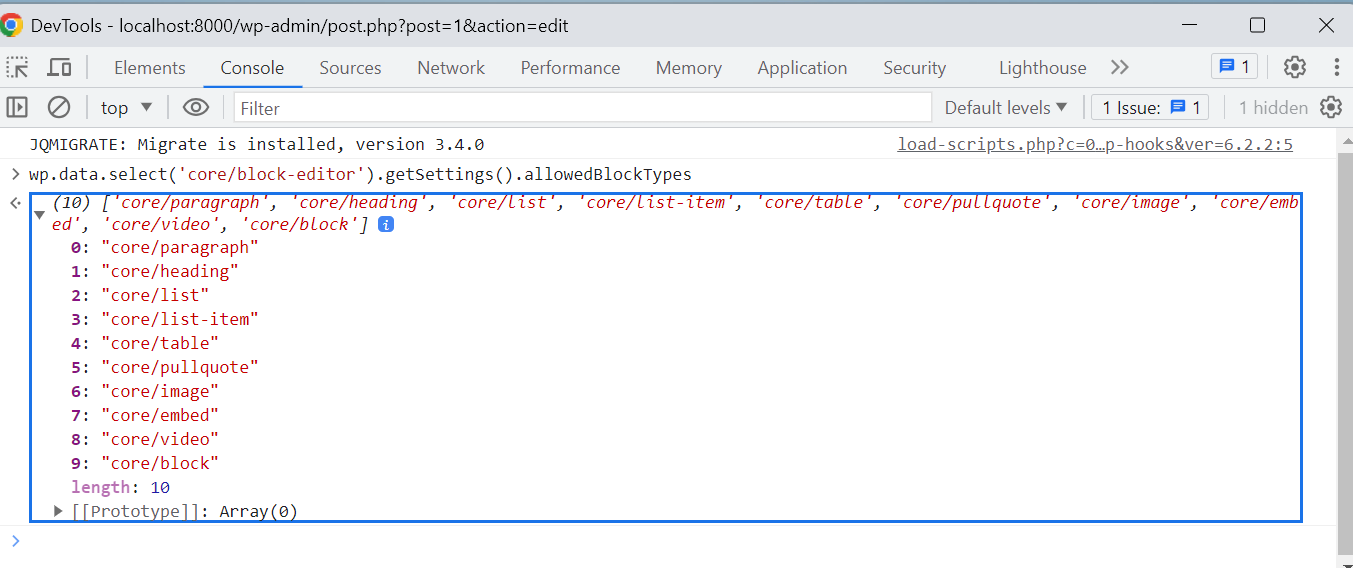
We can also get a list of active blocks being used in the page from the console like this.
wp.data.select('core/block-editor').getBlocks();
Conclusion
By limiting what blocks are availible to the admin users we can help manage the website brand and design. It will also allow you to focus on just the needed blocks for your website when moving forward with customizations.