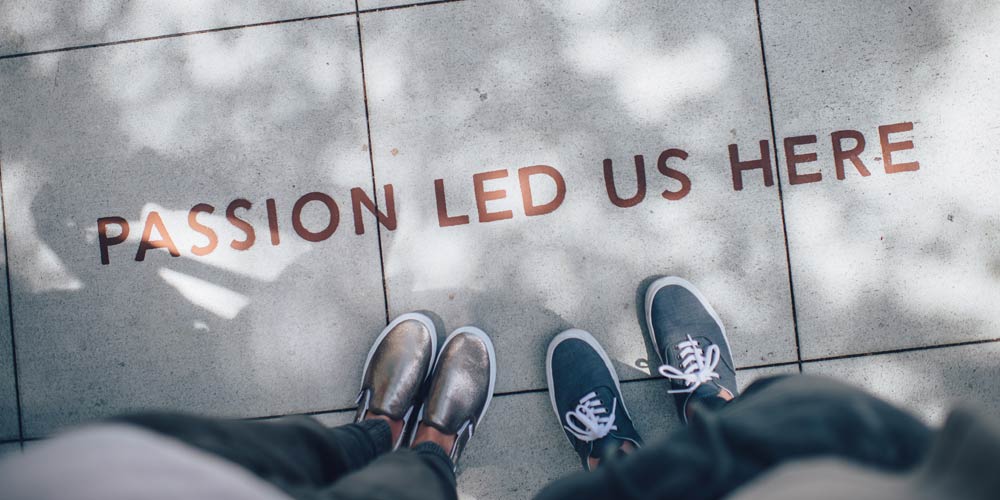
One of the first things I do when starting a new WordPress project is clean out unused code. Out of the box, WordPress adds a bunch of features that we don't typically use, like emojis, RSS feed, embed script, and block styles.
Disabling these extras will give you a cleaner slate to work from, improve loading speeds, and the source code won't look so scary. Keep in mind you will need to be logged out to view the source code results without the admin bar, like what a visitor would be seeing in the DOM.
We can do all of this in our functions.php
file.
Important! Editing the functions.php
file of a WordPress theme could break your site if not done correctly. I recommend having a local environment to test these functions out on before putting them on a live site.
Disable the Emogis
I never use these, so they are the first to go.
//remove emoji support
remove_action('wp_head', 'print_emoji_detection_script', 7);
remove_action('wp_print_styles', 'print_emoji_styles');
This will remove the action that is printing the emoji detection script and all of the emoji styles. The emojis can be useful for things like comments, so if you ever want to add them back you can just comment out the functions.
Disable The RSS Feed For The Blog
Sometimes you're not going to be using the blog, or you don't need to run the blog RSS feed.
// Remove rss feed links
remove_action( 'wp_head', 'feed_links_extra', 3 );
remove_action( 'wp_head', 'feed_links', 2 );
Go to the source page of a blog post to see if the RSS feed link is removed.
Disable The wp-embed Script Link
This script is responsible for converting links into embed frames for players like YouTube and SoundCloud. This script is handy if you are not familiar with HTML and embedding iframes, as it will generate the the embed player for you on page load.
// remove wp-embed
add_action( 'wp_footer', function(){
wp_dequeue_script( 'wp-embed' );
});
We are using the wp_dequeue_script
function to remove the linked javaScript file. You can also write this the following way, by separating the action hook from the function. This goes for any of the hooks below.
function my_deregister_scripts(){
wp_deregister_script( 'wp-embed' );
}
add_action( 'wp_footer', 'my_deregister_scripts' );
Disable The Block and Comment Libraries
If you are not going to be using the Gutenberg Blocks editor, then there is no need to load these styles.
add_action( 'wp_enqueue_scripts', function(){
// // remove block library css
wp_dequeue_style( 'wp-block-library' );
// // remove comment reply JS
wp_dequeue_script( 'comment-reply' );
} );
While we're using the wp_enqueue_scripts
function, we can dequeue other styles or scripts like the comment reply functionality. Yes, you can turn off comments in the admin, although it is good to officially turn it off here.
Disable The Auto-Generated Responsive Images
WordPress creates many different size images to help improve performance across different devices. This can really add to loading times and make the media library a mess. If you do your own custom image optimization for responsive you can disable this feature. This code was provided by Jeff Starr from: Perishable Press
// disable generated image sizes
function shapeSpace_disable_image_sizes($sizes) {
unset($sizes['thumbnail']); // disable thumbnail size
unset($sizes['medium']); // disable medium size
unset($sizes['large']); // disable large size
unset($sizes['medium_large']); // disable medium-large size
unset($sizes['1536x1536']); // disable 2x medium-large size
unset($sizes['2048x2048']); // disable 2x large size
return $sizes;
}
add_action('intermediate_image_sizes_advanced', 'shapeSpace_disable_image_sizes');
// disable scaled image size
add_filter('big_image_size_threshold', '__return_false');
// disable other image sizes
function shapeSpace_disable_other_image_sizes() {
remove_image_size('post-thumbnail'); // disable images added via set_post_thumbnail_size()
remove_image_size('another-size'); // disable any other added image sizes
}
add_action('init', 'shapeSpace_disable_other_image_sizes');
After disabling the auto-generated images, the final step is to disable the srcset
markup that is added to every img
tag on the frontend. The srcset
attribute is added to specify the image file URL to use for the different media
sizes.
// disable srcset on frontend
function disable_wp_responsive_images() {
return 1;
}
add_filter('max_srcset_image_width', 'disable_wp_responsive_images');
Cleaning Up The Function File
These snippets could also be put in a custom plugin. They will have to be managed and kept track of, so it is important to keep them organized. I tend to put each one in its own file in the inc
folder and link them with the get_template_directory()
in function.php like this:
/*
* Include cleanup functions
*/
require get_template_directory() . '/inc/disable_media_duplicates.php';
// Disable srcset
require get_template_directory() . '/inc/disable_srcset_img.php';
// Remove the WordPress Block Styles
require get_template_directory() . '/inc/disable_blocks.php';
// Disable comments
require get_template_directory() . '/inc/disable_comments.php';
// Disable emojis
require get_template_directory() . '/inc/disable_emojis.php';
This way we can easily turn them on and off by commenting them out. This approach to working with WordPress will give you much more control over your custom WordPress website. This is also a good way to keep track of and have control over of your custom developments, while minimizing the amount of site plugins.
Conclusion
As you can see, this is an important part of WordPress development. It is best to add a cleanup process to the beginning of your workflow, so you are not having to chase these features around while you are in the building or managing process.